Solution
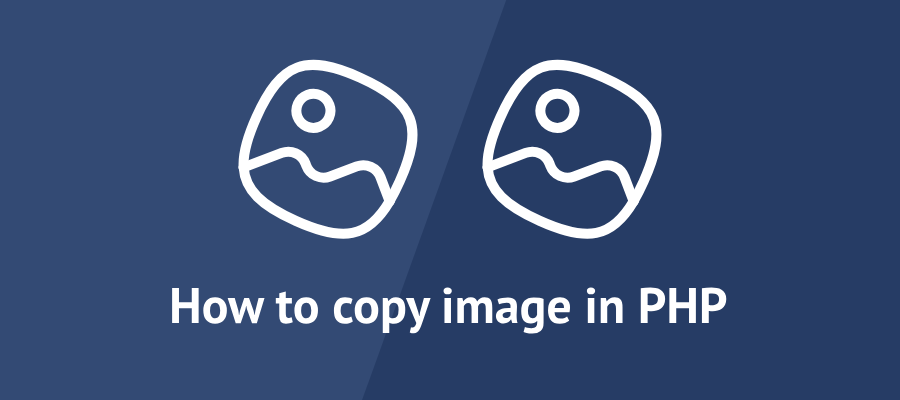
Created 4 month back
How to copy image in PHP
Copying an image in PHP can be achieved using the GD library or the newer and more versatile Imagick
extension. Here are examples using both methods:
// Load the original image
$originalImage = imagecreatefromjpeg('path/to/original.jpg');
// Get the dimensions of the original image
$width = imagesx($originalImage);
$height = imagesy($originalImage);
// Create a new blank image with the same dimensions
$newImage = imagecreatetruecolor($width, $height);
// Copy the original image onto the new image
imagecopy($newImage, $originalImage, 0, 0, 0, 0, $width, $height);
// Save the copied image
imagejpeg($newImage, 'path/to/copied.jpg');
// Free up memory
imagedestroy($originalImage);
imagedestroy($newImage);
Using Imagick Extension:
// Create an Imagick object from the original image
$originalImage = new Imagick('path/to/original.jpg');
// Clone the original image
$newImage = $originalImage->clone();
// Save the cloned image
$newImage->writeImage('path/to/copied.jpg');
// Destroy Imagick objects
$originalImage->destroy();
$newImage->destroy();
Replace 'path/to/original.jpg'
with the path to your original image file and 'path/to/copied.jpg'
with the desired path for the copied image file.
Remember to have the appropriate permissions set for reading the original image and writing the copied image to the filesystem.